Гостевая книга на PHP
05.09.2018
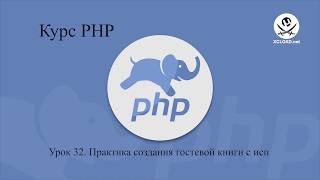
Этот PHP пакет может использоваться для организации и управления гостевой книгой или простым блогом используя базу данных MySQL. Он может создать необходимую таблицу в базе данных MySQL для хранения записей о гостевой книге, которая может также работать как простой блог.
Пакет выполняет несколько действий по управлению гостевыми книгами, такие как: добавление новых записей, отправка сообщений по электронной почте для проверки пользователей добавляющих записи, поиск и отображение записей в гостевой книге, подтверждение или удаление гостевых книг.
Первый проект на PHP. Гостевая книга #2
Лицензия - свободно для образовательных целей.
Исходник основного класса скрипта гостевой книги
Скачать полный архив со скриптом.
abstract class basicIO{ public $database = 'database'; public $user = 'user'; public $password = 'password'; public $databaseserver = 'localhost'; public $webserver = 'localhost'; abstract function displayResults($results); public function __construct(){ session_start(); error_reporting(0); } public function createDB(){ // this is just so you have to right db, table and user rights. $sql = 'CREATE DATABASE IF NOT EXISTS '.$this->database; mysql_query($sql); $sql = 'CREATE TABLE IF NOT EXISTS guestbook'; $sql .= '('; $sql .= ' PK_GuestbookID INT AUTO_INCREMENT, '; $sql .= ' name VARCHAR(50), '; $sql .= ' text TEXT, '; $sql .= ' headline VARCHAR(200), '; $sql .= ' imgurl VARCHAR(50), '; $sql .= ' email VARCHAR(50), '; $sql .= ' approved INT(1) UNSIGNED ZEROFILL, '; $sql .= ' verified INT(1) UNSIGNED ZEROFILL, '; $sql .= ' date DATETIME, '; $sql .= ' PRIMARY KEY(PK_GuestbookID)'; $sql .= ') ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8 '; mysql_query($sql); $sql = "GRANT ALL ON ".$this->database.".* TO '".$this->user."'@'".$this->databaseserver."' identified by '".$this->password."'"; mysql_query($sql); return 1; } public function destroyTables(){ $sql = "DROP TABLE IF EXISTS guestbook"; mysql_query($sql); return 1; } public function connectDB(){ if($_SERVER['HTTP_HOST'] == 'localhost'){ $con = mysql_connect('localhost',$this->user,$this->password); } else { $con = mysql_connect($this->databaseserver,$this->user,$this->password); } if (!$con){ die('Could not connect: ' . mysql_error()); $con = 0; } else { mysql_select_db($this->database, $con); } return $con; } public function closeDB($con){ if($con) mysql_close($con); } } class guestbookUser extends basicIO{ public $name; public $text; public $imgURL; public $email; public $id; public $con; public function __construct(){ $this->id = 0; $this->name = ''; $this->text = ''; $this->imgURL = ''; $this->email = ''; $this->headline = ''; $this->con = self::connectDB(); } public function displayResults($results){ $result = ''; foreach ($results as $res){ list($id,$name,$text,$headline,$imgurl,$email,$date) = $res; $result .= '<table border="1" width="600">'; $result .= '<tr><td colspan="2" align="left" width="200">'.$headline.' </td></tr>'; $result .= '<tr><td valign="top" align="center">'; if($imgurl == ''){ $img = 'user.jpg'; } else { $img = $imgurl; } $result .= '<img src="'.$img.'" width="160" /><br />'; $result .= 'By: '.$name.'<br />On: '.$date; $result .= '</td><td valign="top" align="left" width="400">'; $result .= $text; $result .= '</td></tr>'; $result .= '</table><br />'; } return $result; } public function readAllguestbook(){ $return = array(); $sql = "SELECT * FROM guestbook WHERE approved = 1 AND verified = 1 ORDER BY PK_GuestbookID DESC"; $result = mysql_query($sql); try{ if(!$result){ throw new Exception("Could not read guestbook!"); } else { while($row = mysql_fetch_array($result)){ $return[] = array($row['PK_GuestbookID'], $row['name'], $row['text'], $row['headline'], $row['imgurl'], $row['email'], $row['date']); } } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $return; } public function sendMailToUser(){ $to = $this->email; // subject $subject = 'Please verify your guestbook entry'; // message $message = ' <html> <head> <title>Need to verify your guestbook entry</title> </head> <body> <p>You need to verify your guestbook entry <br /> Please click the link below <br /> <a href="http://'.$this->webserver.'/guestbook/verifyguestbook.php?entry='.$this->id.'&email='.$this->email.'">Verify your guestbook</a><br /> </p> </body> </html>'; // To send HTML mail, the Content-type header must be set $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers .= 'From: guestbook <[email protected]>' . "\r\n"; // Mail it mail($to, $subject, $message, $headers); } public function verifyguestbookEntry($id,$email){ $result = 0; $sql = "UPDATE guestbook SET verified = 1 WHERE email = '".$email."' AND PK_GuestbookID = ".$id; try{ if(!mysql_query($sql)){ throw new Exception("Could not approve guestbook entry on ID: ".$id); } else { $result = $id; } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $result; } public function createguestbookEntry(){ $result = 0; $sql = "INSERT INTO guestbook (name, text, headline, imgurl, email, approved, verified, date) VALUES ('".$this->name."','".$this->text."','".$this->headline."','".$this->imgURL."','".$this->email."',0,0,NOW())"; try{ if(!mysql_query($sql)){ throw new Exception("Could not create new guestbook entry "); } else { $sql = "SELECT PK_GuestbookID FROM guestbook ORDER BY PK_GuestbookID DESC LIMIT 1"; $row = mysql_fetch_array(mysql_query($sql)); try{ if(!$row){ throw new Exception("Could not find newest guestbook entry"); } else { $this->id = $row['PK_GuestbookID']; echo $this->id; self::sendMailToUser(); $result = 1; } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $result; } public function __destruct(){ $this->id = 0; $this->name = ''; $this->text = ''; $this->imgURL = ''; $this->email = ''; $this->headline = ''; self::closeDB($this->con); } } class guestbookAdmin extends basicIO{ public $name; public $email; public $id; public $con; public function __construct(){ $this->id = 0; $this->name = ''; $this->email = ''; $this->con = self::connectDB(); } public function displayResults($results){ $result = '<table border="1">'; foreach ($results as $res){ $result .= '<tr>'; foreach($res as $final){ $result .= '<td>'.$final.'<td>'; } $result .= '</tr>'; } $result .= '</table>'; return $result; } public function readAllguestbook($approved){ $return = array(); $sql = "SELECT * FROM guestbook WHERE approved = ".$approved; $result = mysql_query($sql); try{ if(!$result){ throw new Exception("Could not read guestbook!"); } else { while($row = mysql_fetch_array($result)){ if(!$approved){ $approve = '<a href="admin.php?entry='.$row['PK_GuestbookID'].'&email='.$row['email'].'&approve=1&guestbook=1">Approve</a>'; } else { $approve = ''; } $delete = '<a href="admin.php?entry='.$row['PK_GuestbookID'].'&email='.$row['email'].'&del=1&guestbook=1">Delete</a>'; $read = '<a href="javascript:readGuestbook('.$row['PK_GuestbookID'].')">Read</a>'; $verified = 'not verified'; if($row['verified']) $verified = 'Verified'; $return[] = array($row['PK_GuestbookID'], $row['name'], $row['email'], $verified , $approve, $delete, $read); } } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $return; } public function readSingleGuestbook($id){ $sql = "SELECT * FROM guestbook WHERE PK_GuestbookID = ".$id; $result = mysql_query($sql); try{ if(!$result){ throw new Exception("Could not read guestbook!"); } else { while($row = mysql_fetch_array($result)){ $return = array($row['PK_GuestbookID'], $row['name'], $row['headline'], $row['text'], $row['imgurl'], $row['date']); } } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $return; } public function approveGuestbook($id,$email){ $result = 0; $sql = "SELECT approved FROM guestbook WHERE email = '".$email."' AND PK_GuestbookID = ".$id." ORDER BY PK_GuestbookID LIMIT 1"; $row = mysql_fetch_array(mysql_query($sql)); try{ if(!$row){ throw new Exception("Could not find guestbook entry"); } else { $sql = "UPDATE guestbook SET approved = 1 WHERE email = '".$email."' AND PK_GuestbookID = ".$id; try{ if(!mysql_query($sql)){ throw new Exception("Could not approve guestbook entry on ID: ".$id); } else { $result = $id; } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $result; } public function destroyGuestbook($id,$email){ $result = 0; $sql = "SELECT PK_GuestbookID FROM guestbook WHERE email = '".$email."' AND PK_GuestbookID = ".$id." ORDER BY PK_GuestbookID LIMIT 1"; $row = mysql_fetch_array(mysql_query($sql)); try{ if(!$row){ throw new Exception("Could not find guestbook entry"); } else { $sql = "DELETE FROM guestbook WHERE email = '".$email."' AND PK_GuestbookID = ".$id; try{ if(!mysql_query($sql)){ throw new Exception("Could not delete guestbook entry on ID: ".$id); } else { $result = $id; } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } } } catch (Exception $e){ echo 'Exception caught: ', $e->getMessage(), "\n"; } return $result; } public function __destruct(){ $this->id = 0; $this->name = ''; $this->email = ''; self::closeDB($this->con); } }Системные требования скрипта:
PHP 5.x и БД MySQL.